Web Serial Api – Arduino
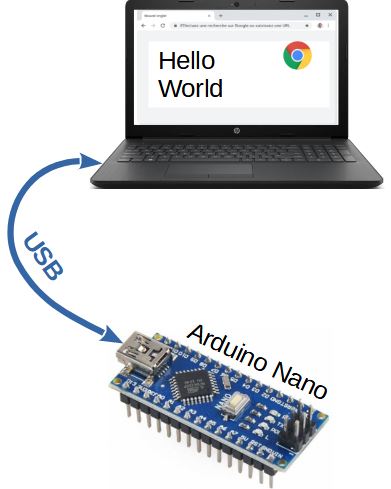
The Serial Web API provides a way for websites to read and write to serial devices. These devices can be connected through a serial port, or be USB or Bluetooth devices that emulate a serial port.
Here we will study a very simple case of an Arduino Nano sending a message to a web page..
Arduino Software
It is a small program that runs on all versions of Arduino with a serial port. It sends the phrase “Hello World” every second. You must use the Arduino IDE to write and download the program. It is not described here, as there are many tutorials on the web.
/*
Serial Print
*/
void setup() {
// initialize serial communication at 9600 bits per second:
Serial.begin(9600);
}
// the loop routine runs over and over again forever:
void loop() {
Serial.println("Hello");
delay(300);
Serial.println("World");
delay(300);
Serial.println("");
delay(300); // delay 300 ms
}
Web client Software
Using a text editor like Notepad ++, you have to write a “WebSerial.html” web page. It can be saved to a local folder. A web server is not necessary. We launch the page in a modern browser like “Chrome”.
<!DOCTYPE html>
<html>
<head>
<meta http-equiv="X-UA-Compatible" content="IE=edge,chrome=1" />
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta name = "author" content = "André Buhart F1ATB">
<title>Web Serial Api</title>
<script>
var port;
var lineBuffer = '';
async function getReader() {
var port = await navigator.serial.requestPort({});
await port.open({ baudRate: 9600 });
const appendStream = new WritableStream({
write(chunk) {
lineBuffer += chunk;
var lines = lineBuffer.split('n');
while (lines.length > 1) {
var message=lines.shift();
console.log(message);
document.getElementById("info").innerHTML=message;
}
lineBuffer = lines.pop();
}
});
port.readable
.pipeThrough(new TextDecoderStream())
.pipeTo(appendStream);
}
function listSerial(){
if (port) {
port.close();
port = undefined;
}
else {
console.log("Look for Serial Port")
getReader();
}
}
</script>
</head>
<body>
<button onclick="listSerial();">Connect</button>
<div id="info"></div>
</body>
</html>
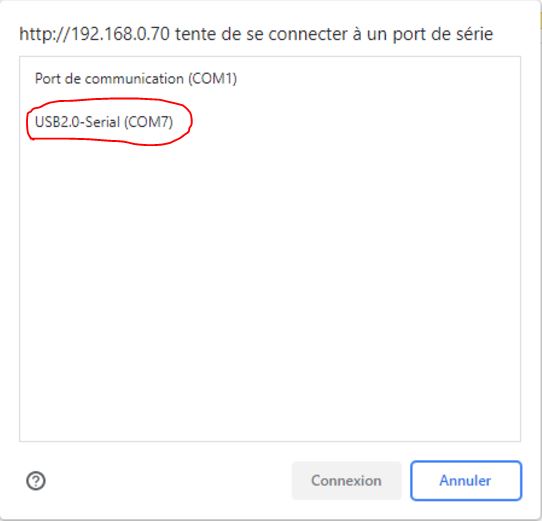
The key to this application in Javascript is the asynchronous function “async function getReader ()” which allows listing serial devices and to connect at 9600 bauds. When the page opens, press “Connect” to display the list of devices visible to your PC and select the one corresponding to the Arduino. If there is a problem, disconnect and reconnect the Arduino. The message is displayed on the web page and the browser console which can be opened by Shift-Ctrl-I.
Now you are free to develop an Arduino-based application. A web page, even locally, can provide a good command interface to manage a project. Of course, it is possible to send commands to the Arduino. You should refer to the online documentation around the “Web Serial API”.
Hi Andre! When the script is running, I get an error in the line
await port.open ({baudRate: 9600});
Error text:
WebSerial.html: 16 Uncaught (in promise) DOMException: Failed to execute ‘open’ on ‘SerialPort’: The port is already open.
I am using Google Chrome version 95.0.4638.69 in Debian-11, I think that the browser does not have permission to access the port
How can this problem be solved?
If the port is already open, force the closing by disconnecting the arduino and then reconnect it. Often, this situation appears because you have previously upload the arduino software with the Arduino IDE which occupies the port.
73
André