Orange PI GPIO
Orange Pi Single Board Computer, similar to the famous Raspberry Pi, offer interesting characteristics, given the diversity of models offered.
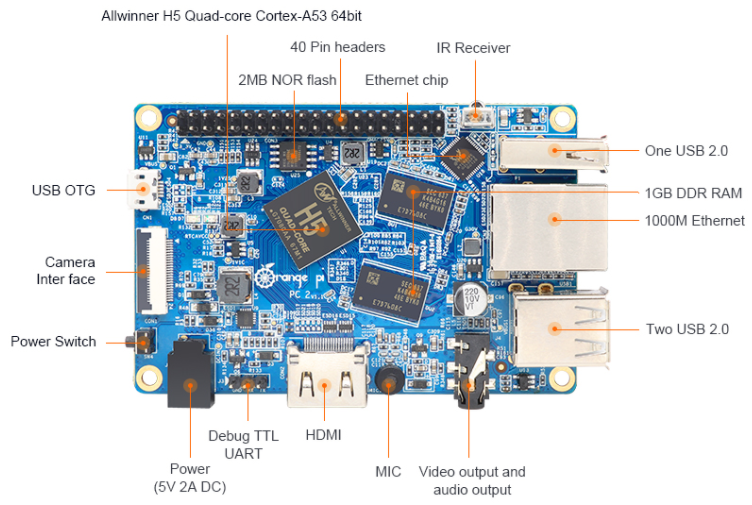
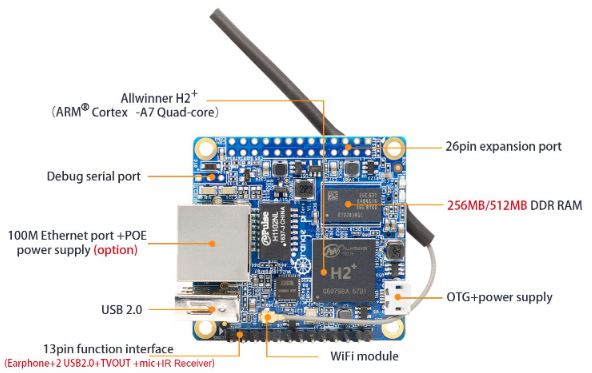
To access the GPIO of an Orange PI, you can use one of the libraries like OPI.Gpio. Unfortunately it does not cover all Orange PI card models. An alternative solution is the Sysfs virtual file system which allows system commands to activate the GPIO pins.
Orange Py PC2 H5 Connector
The PC2 H5 card has a 40-pin connector according to the diagram below.
To attack one of the input or output pins, you have to find the reference of the corresponding GPIO. The table below is valid for the Orange Pi PC2 H5. So if you want to use the physical pin 40, it corresponds to the GPIO 199.
GPIO | Function | Pin | Pin | Function | GPIO |
3.3V | 1 | 2 | 5V | ||
12 | PA12 (TWI0_SDA/DI_RX/PA_EINT12) | 3 | 4 | 5V | |
11 | PA11 (TWI0_SCK/DI_TX/PA_EINT11) | 5 | 6 | GND | |
6 | PA6 (SIM_PWREN/PWM1/PA_EINT6) | 7 | 8 | PC5 (NAND_RE/SDC2_CLK) | 69 |
GND | 9 | 10 | PC6 (NAND_RB0/SDC2_CMD) | 70 | |
1 | PA1 (UART2_RX/JTAG_CK0/PA_EINT1) | 11 | 12 | PD14 (RGMII_NULL/MII_TXERR/RMII_NULL) | 110 |
0 | PA0 (UART2_TX/JTAG_MS0/PA_EINT0) | 13 | 14 | GND | |
3 | PA3 (UART2_CTS/JTAG_DI0/PA_EINT3) | 15 | 16 | PC4 (NAND_CE0) | 68 |
3.3V | 17 | 18 | PC7 (NAND_RB1) | 71 | |
15 | PA15 (SPI1_MOSI/UART3_RTS/PA_EINT15) | 19 | 20 | GND | |
16 | PA16 (SPI1_MISO/UART3_CTS/PA_EINT16) | 21 | 22 | PA2 (UART2_RTS/JTAG_DO0/PA_EINT2) | 2 |
14 | PA14 (SPI1_CLK/UART3_RX/PA_EINT14) | 23 | 24 | PA13 (SPI1_CS/UART3_TX/PA_EINT13) | 13 |
GND | 25 | 26 | PA21 (PCM0_DIN/SIM_VPPPP/PA_EINT21) | 21 | |
19 | PA19 (PCM0_CLK/TWI1_SDA/PA_EINT19) | 27 | 28 | PA18 (PCM0_SYNC/TWI1_SCK/PA_EINT18) | 18 |
7 | PA7 (SIM_CLK/PA_EINT7) | 29 | 30 | GND | |
8 | PA8 (SIM_DATA/PA_EINT8) | 31 | 32 | PG8 (UART1_RTS/PG_EINT8) | 200 |
9 | PA9 (SIM_RST/PA_EINT9) | 33 | 34 | GND | |
10 | PA10 (SIM_DET/PA_EINT10) | 35 | 36 | PG9 (UART1_CTS/PG_EINT9) | 201 |
107 | PD11 (RGMII_NULL/MII_CRS/RMII_NULL) | 37 | 38 | PG6 (UART1_TX/PG_EINT6) | 198 |
GND | 39 | 40 | PG7 (UART1_RX/PG_EINT7) | 199 |
Orange Pi Zero H2 Connector
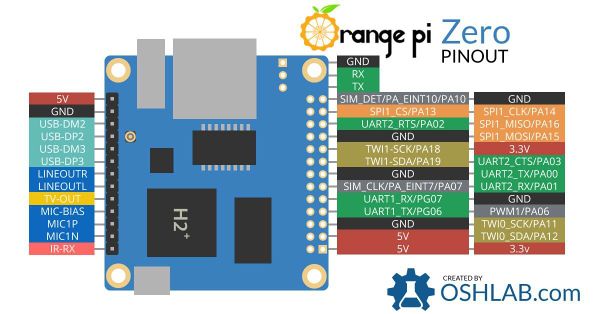
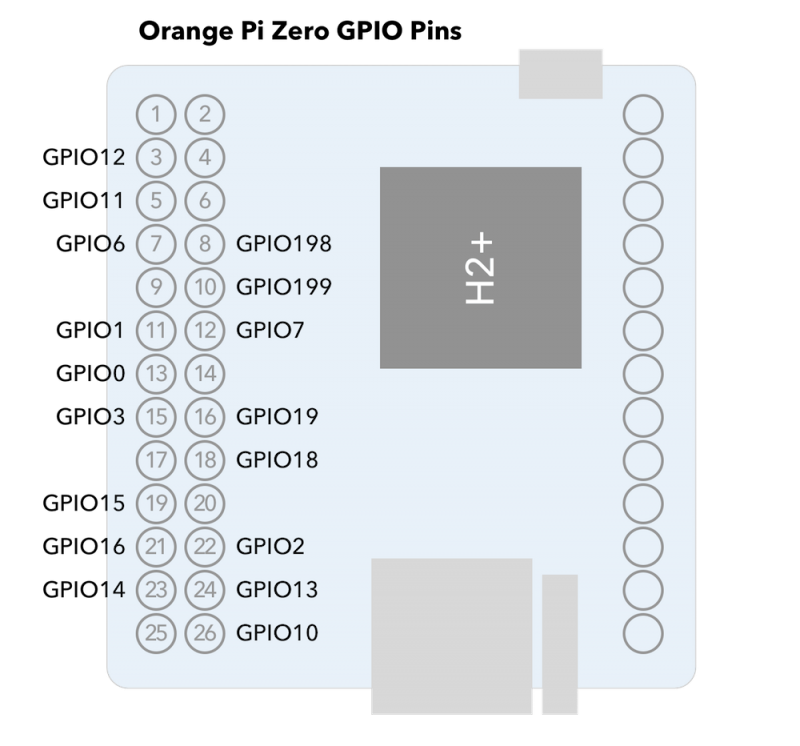
Example Oscillator
For a given pin, there are 3 references:
- the pin number: 1,2,3…
- the functional name: PA00,PA01…
- the GPIO reference: GPIO1, GPIO199….
With the Sysfs virtual files method, you need to know the GPIO number of the pin you want to activate. To do this, search the web for a diagram or a correspondence table.
Below is an oscillator program in python 3. It activates the GPIO199 which corresponds to pin 40 of an Orange Pin PC2 H5 or pin 10 of an Orange Pi Zero H2. Attention, important constraint. You must be root to access the GPIO.
#!/usr/bin/python3 Python 3 environment #-- coding: utf-8 -- import os # To execute system command from time import sleep # this lets us have a time delay numgpio=199 numgpio=str(numgpio) print ("numgpio:"+numgpio) os.system("echo "+numgpio+" > /sys/class/gpio/export") os.system("echo out > /sys/class/gpio/gpio"+numgpio+"/direction") try: print ("Square signal around 50Hz . Press CTRL+C to exit") while True: os.system("echo 0 > /sys/class/gpio/gpio"+numgpio+"/value") sleep(0.01) os.system("echo 1 > /sys/class/gpio/gpio"+numgpio+"/value") sleep(0.01) except KeyboardInterrupt: # set port/pin value to 0/LOW/False os.system("echo 0 > /sys/class/gpio/gpio"+numgpio+"/value") # Clean GPIO os.system("echo "+numgpio+" > /sys/class/gpio/unexport") print ("Bye from F1ATB.")
to start it, if the program( test.py) is on your desktop:
python3 /home/user/Desktop/test.py
You can enter commands manually from a terminal window.
The …export command activates GPIO 199 which corresponds to pin 40.
The direction command, here out to be output.
The value commands alternately 0 or 1 to make an oscillator.
Then in case of Ctrl-C, we finish properly by releasing the GPIO 199.prement en libérant le GPIO 199.
To overcome the root problem, one solution may be to launch the program when starting up the Orange PI. Switch to root (sudo su) and open the cron jobs file by typing:
crontab -e
Put a line at the bottom of the file:
@reboot python3 /home/xxx/test.py
/home/xxx is the folder where your test.py file is located.
Summary of Sysfs Commands
On Armbian, you see the GPIO hardware exposed in the filesystem under /sys class/gpio.
$ ls /sys/class/gpio export gpio199 gpiochip0 gpiochip352 unexport
There are the gpio currently used and the GPIO controllers noted “gpiochip”.
The basic steps to use a GPIO pin (here the 199 or pin 40 or 10) from the sysfs interface are as follows:
Activation Gpio199 :
$ echo 199> /sys/class/gpio/export
Pin as input:
$ echo in> /sys/class gpio/gpio199 direction
Pin as output:
$ echo out> /sys /class/gpio/gpio199/direction
Replaying the file to verify the current status :
$ cat /sys/class/gpio/gpio199/direction
Output state:
$ echo 0> /sys/class/gpio/gpio199/value
or
$ echo 1> /sys/class/gpio/gpio199/value
Reading value in input:
$ cat /sys/class/gpio/gpio199/value
Freeing Gpio:
If you are done using the GPIO pin, just write the pin name to the export file.
$ echo 199> /sys/class/gpio/unexport
The entry for gpio199 will no longer appear in sysfs:
$ ls/sys/class/gpio
Access with Python
The “shell” commands can be used in an application coded in python.
To issue a command such as the activation of a GPIO pin:
os.system(“echo 199> /sys/class/gpio/export”)
To read a state of a gpio pin, we will use file reading:
fichier=open("/sys/class/gpio/gpio199/value","r")
r=fichier.read()
fichier.close()
Beware of activating an output pin attacking a relay that goes “on” with a zero input. The latter will be stuck for a few ms between the passage of the output pin “out” and its setting to 1. To avoid this inconvenience, pass the 2 commands “out” and “1” to the system in a single instruction.
os.system("echo out > /sys/class/gpio/gpio199/direction ; echo 1 > /sys/class/gpio/gpio199/value")
Remarks
- A pin configured as an output can be read as input to know its state.
- You cannot pull up or down (pullup, puldown) an entry.
- This very general solution is applicable to all Orange Pi
Recent Comments